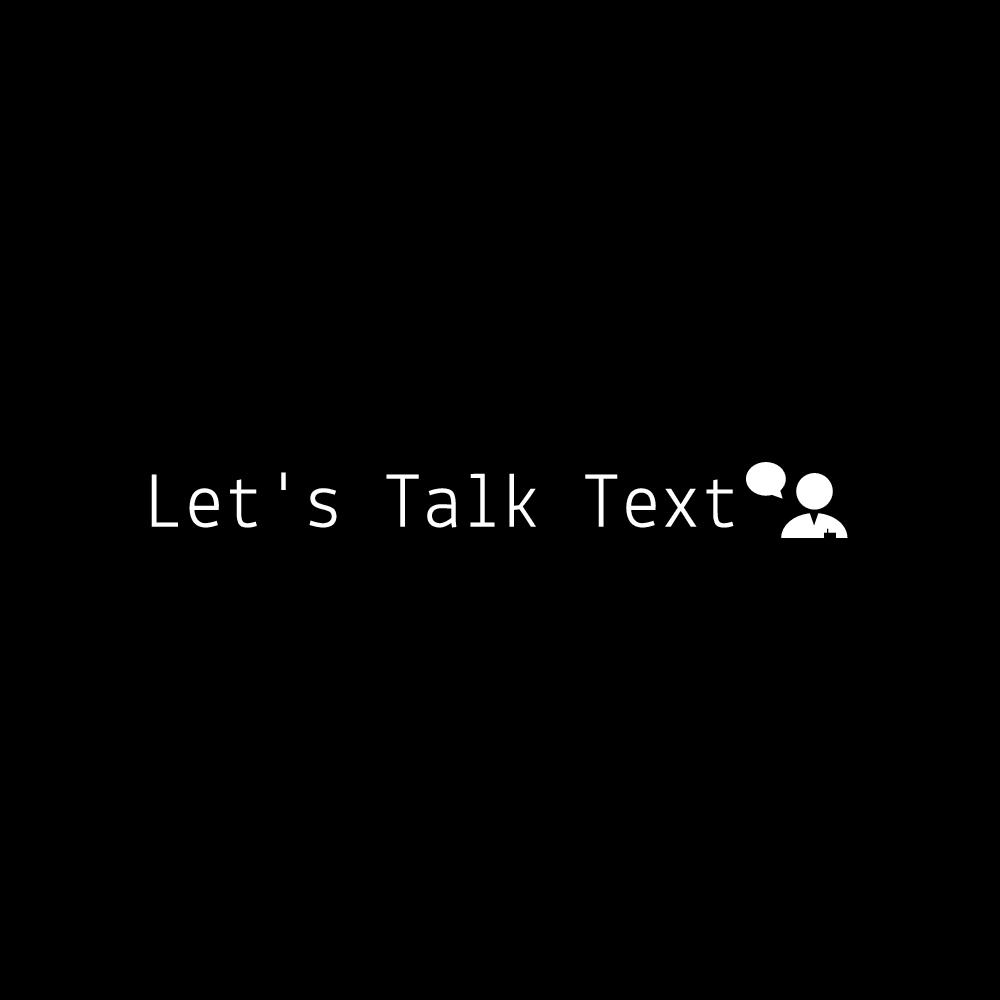
Let's Talk Text
Join hundreds of other subscribers and keep up to date with developments in ML and NLP. Comes out every Wednesday at 8am.
Join hundreds of other subscribers and keep up to date with developments in ML and NLP. Comes out every Wednesday at 8am.